Day03 手部關鍵點檢測 Hand Landmarks Detection
🟡 先看看程式的效果
今天的程式是利用 OpenCV 和 MediaPipe 來進行即時的手部偵測和關鍵點繪製, 是這類手部偵測程式的基本型, 之後連續幾天會建基於這個程式逐步增加不同的功能。
Today’s program uses OpenCV and MediaPipe for real-time hand detection and keypoint drawing. It serves as a basic model for hand detection programs. Over the next few days, various features will be gradually added to this program.
🟡 學習目標
認識及使用 OpenCV
OpenCV,全名是 Open Source Computer Vision Library,是一個開源的計算機視覺和機器學習軟體庫。OpenCV 提供了數以千計的演算法,這些演算法可以用來進行影像處理、物體識別、運動追蹤、人臉識別等多種計算機視覺任務。
認識及使用 MediaPipe
MediaPipe 是由 Google 提供的一個開源框架,用於構建多模態機器學習管道。它特別適合處理和分析視覺數據,如視頻和圖像。MediaPipe 提供了許多現成的解決方案和工具,能夠幫助開發者輕鬆實現手勢識別、面部偵測、姿態估計等功能。
🔻以下是 Mediapipe 對手部辨識的 Landmarks 以及其編號。
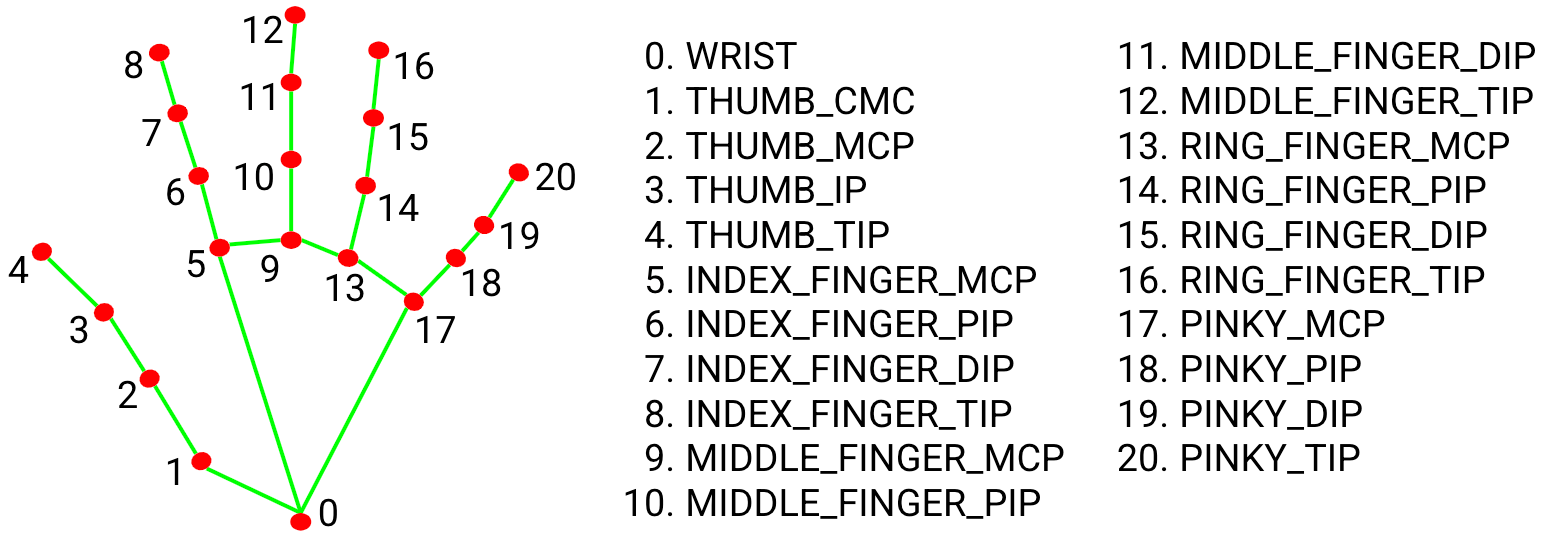
🟡 程式碼
請先下載 “Hand Landmarks Detection 手部關鍵點檢測.py”
請按此下載
'''
Python + AI in 21 days
https://jasonworkshop.com/python
Designed by Jason Workshop
[請勿修改以上內容]
---
預備工作:
首先,請確保已安裝 opencv-python, mediapipe 模組
如不確定可直接在 Windows 的 cmd prompt 執行以下指定
pip install opencv-python mediapipe
'''
import cv2
import mediapipe as mp
# 初始化 MediaPipe Hands 模組
mp_hands = mp.solutions.hands
hands = mp_hands.Hands(static_image_mode=False, max_num_hands=2, min_detection_confidence=0.7)
# static_image_mode:是否靜態模式,若為 True 則每張圖像都會獨立處理
# max_num_hands:最多手部偵測數量
# min_detection_confidence:手部檢測的最低可信度
mp_drawing = mp.solutions.drawing_utils # 繪製工具
# 開啟攝影機
cap = cv2.VideoCapture(0)
while cap.isOpened():
ret, frame = cap.read() # 讀取攝影機畫面
if not ret:
print("無法讀取攝影機畫面")
break
# 轉換 BGR 圖像到 RGB
rgb_frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
# 偵測手部
result = hands.process(rgb_frame)
# 繪製手部關鍵點和連線
if result.multi_hand_landmarks:
for hand_landmarks in result.multi_hand_landmarks:
mp_drawing.draw_landmarks(
frame,
hand_landmarks,
mp_hands.HAND_CONNECTIONS,
landmark_drawing_spec=mp_drawing.DrawingSpec(color=(0, 255, 0), thickness=2, circle_radius=2), # 關鍵點樣式
connection_drawing_spec=mp_drawing.DrawingSpec(color=(255, 255, 255), thickness=1, circle_radius=2) # 連線樣式
)
# 顯示圖像
cv2.imshow('Hand Landmarks Detection', frame)
# 按下 ESC 鍵退出
if cv2.waitKey(1) & 0xFF == 27:
break
# 釋放攝影機並關閉所有視窗
cap.release()
cv2.destroyAllWindows()
🟡 小小挑戰一下
大家可以嘗試進行一些修改或改良喔! 例如:
✌️修改 Landmark 上點和線的顔色。
😁 明天見!